Azure Pipelines and Dependabot
Keeping dependencies up to date using Dependabot and Azure Pipelines
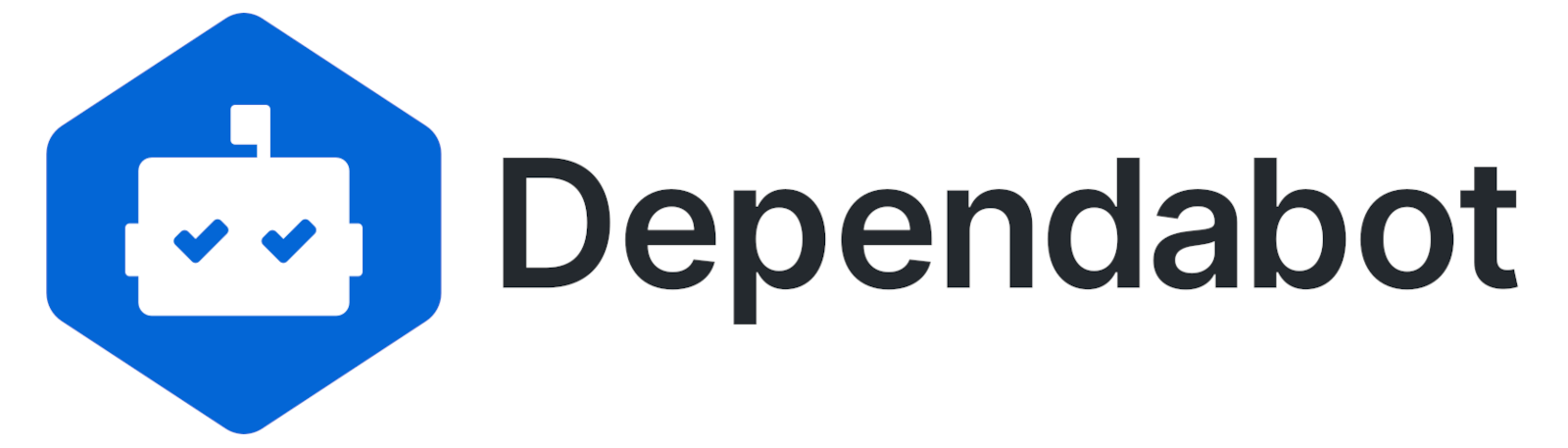
Keeping your dependencies up to date in a project is a really easy way to try and keep the software secure. New releases of a dependency often include
- Patches for security vulnerabilities!
- Performance improvements!
- Awesome new features!
- Bug fixes!
It can also be quite a boring activity and can be time-consuming for a team maintaining the project to run updates regularly into production. Fortunately tools like Dependabot exist!
Dependabot?
Dependabot creates pull requests on your repos with the dependencies you should update. You can read about how it works ; but in a nutshell
- it checks for updates of your dependencies
- it opens up a pull request on your repo
- you review the PR and merge
This is an awesome tool to save you time and I find it makes keeping your dependencies up to date really easy.
Integration with Azure Pipelines
Dependabot is baked into the GitHub ecosystem and really easy to use there. Recently I needed to solve this problem on a project in Azure DevOps using Azure Pipelines and thought I would share my solution.
If you search the Azure DevOps Extension Marketplace for Dependabot you will find this extension made by Tingle Software . It is always great to find extensions to speed up integration and I gave this one a whirl.
The extension is feature rich and works really well with little configuration required. Simply add the below to an Azure Pipeline and run it, and you’ll end up with a host of PR’s!
|
|
Have a look at all the Task Parameters
that the extension supports to fine tune your implementation. The above checks for nuget
dependencies on the main
branch, limits the number of PR’s raise to be 10 and sets the PR to autocomplete (letting our branch policies and PR validation pipelines perform all their checks).
Work Item Linking
In the project I’m working in we have a policy set on all PR’s to ensure they are linked to a work item. When I ran the above pipeline, I ended up with 10 PR’s, none of which were linked to a work item so I couldn’t quickly review and merge each one. The extension handles this by allowing you to pass in a workItemId
parameter.
workItemId
parameter has been renamed
to milestone
so please be on the look out for this change!The Microsoft team have an extension for creating work items which is really configurable. For my teams workflow, we wanted to have a User Story on our board with all the PR’s linked to it. We also didn’t want the pipeline creating duplicate User Stories every time it runs if we already had one open that we are working on. After a bit of trial and error, adding the below to the pipeline gave us the desired result.
|
|
Docker Caching
The Dependabot extension
depends on a Docker image hosted on Docker Hub
. The image is around 4.4GB
in size and can take some time to download in the pipeline.
In the docs it mentions
Since this task makes use of a docker image, it may take time to install the docker image. The user can choose to speed this up by using Caching for Docker in Azure Pipelines.
The caching tasks can look a bit confusing, breaking it down you need 3 parts:
|
|
This checks if there is a cached version of the image to be used. If yes, DOCKER_CACHE_HIT
is set to true
, otherwise false
.
|
|
If we have a cached version of the image, we need to pipeline to download it. This step only runs if DOCKER_CACHE_HIT
is set to true
and will download the cached archive and load it into the docker context.
|
|
If we do not have a cached version of the image we need to pull it down from Docker Hub and save it as an archive to be cached.
Finishing up
We have a pipeline that runs on a schedule, running all the above steps together. A complete example can be found on GitHub here .
|
|
In summary the pipeline does the following for you
- Runs on a weekly schedule (update the cron to suite your needs)
- Creates a new work item, with the tags we would like (avoiding duplicates)
- Tries to use a cached version of the image for faster builds (creating a cached version if not found)
- Run Dependabot, limiting the number of open PR’s to 10, linking the PR’s to the created work item and completing the PR once all the policies pass
Hopefully this will help you to get your projects running in Azure DevOps nice and up to date!